* Annotation
@RestController
RESTful 웹 서비스의 컨트롤러임을 선언.
@RequestMapping
요청을 특정 컨트롤러 클래스나 메서드에 매핑할 때 사용.
@GetMapping
HTTP GET 요청을 특정 핸들러 메서드에 매핑할 때 사용. 즉, 지정된 URL의 GET 요청을 처리하는 메서드를 정의한다.
@PathVariable
URL 경로의 일부를 메서드 매개변수로 전달받을 때 사용. 즉, URL 패턴 중 일부를 동적으로 받아서 사용할 수 있다.
@RequestParam
HTTP 요청의 쿼리 파라미터를 메서드의 매개변수로 바인딩할 때 사용.
요청 파라미터의 이름과 메서드 매개변수의 이름이 일치해야, name 속성을 사용하여 다른 이름의 파라미터를 바인딩할 수도 있다.
* 실습 세팅
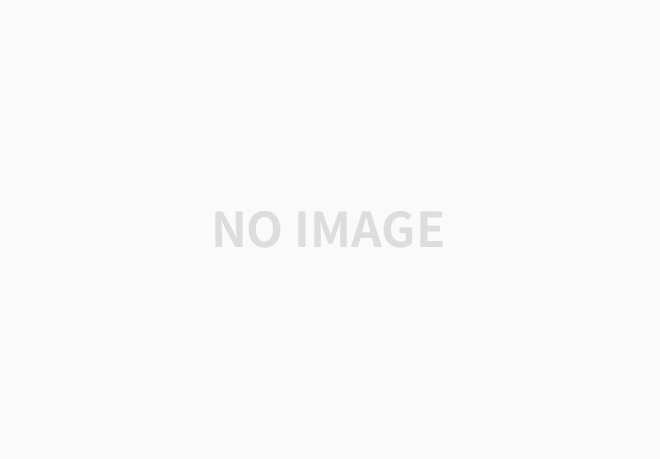
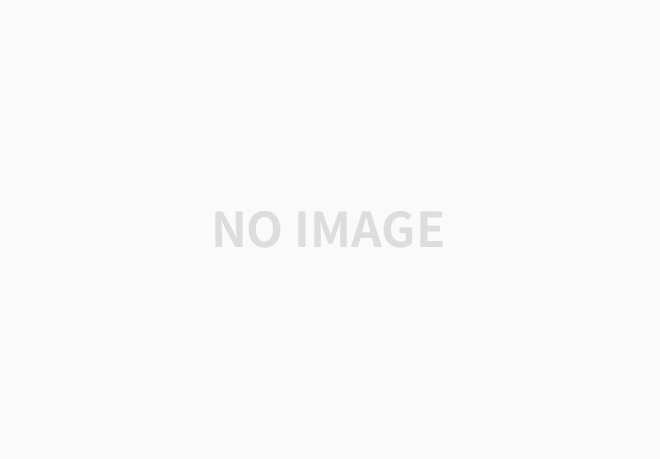
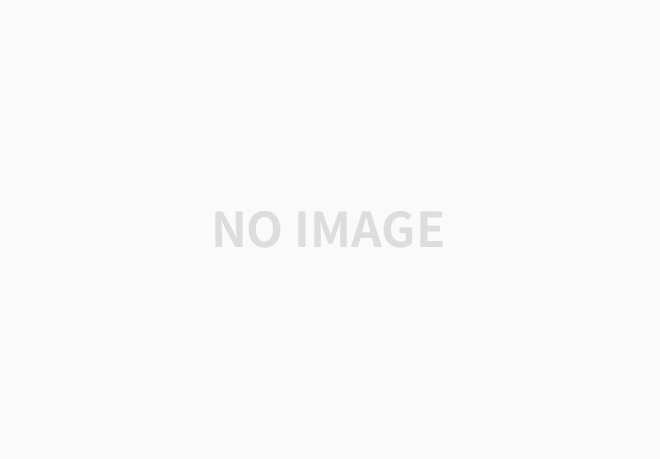
실습 1
package com.example.rest_api.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController // 이 클래스는 RESTful 서비스의 컨트롤러임을 나타냄
@RequestMapping("/api") // api로 시작하는 주소는 이 컨트롤러로 요청을 받음
// 특정 주소 api에 hello라는 주소가 요청이 들어오면, 이 메서드로 매핑
public class RestApiController {
@GetMapping(path = "/hello") // hello라는 값을 요청하면 여기서 특정 문자를 리턴
public String hello(){
return "Hello Spring Boot";
}
}
@RestController: 이 클래스가 RESTful 서비스의 컨트롤러임을 나타냄
@RequestMapping("/api"): 이 컨트롤러는 /api로 시작하는 모든 요청을 처리
@GetMapping(path = "/hello"): GET 메서드로 /api/hello 주소로 요청이 들어오면 hello() 메서드가 호출됩
public String hello(): hello() 메서드는 "Hello Spring Boot" 문자열 반환
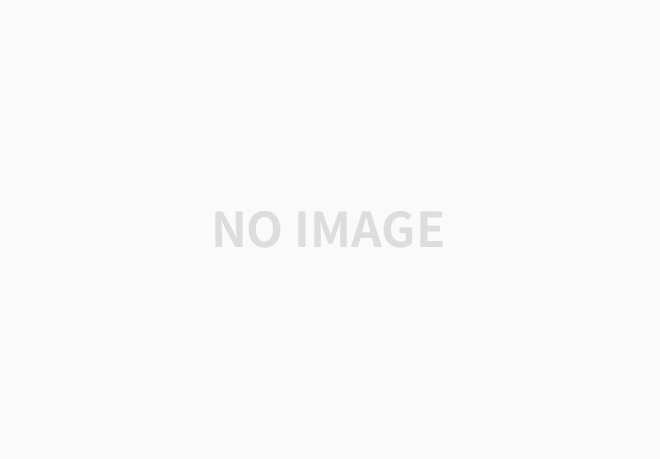
실습 2
package com.example.rest_api.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController // 이 클래스는 RESTful 서비스의 컨트롤러임을 나타냄
@RequestMapping("/api") // "/api"로 시작하는 모든 요청은 이 컨트롤러에서 처리됩니다.
public class RestApiController {
// HTTP GET 요청이 "/api/hello" 경로로 들어오면 이 메서드가 처리
@GetMapping(path = "/hello")
public String hello(){
var html = "<html> <body> <h1> Hello Spring Boot </h1> </body> </html>";
return html; // "Hello Spring Boot" 문자열을 HTML 형식으로 반환
}
}
/api/hello 경로로 GET 요청을 하면 hello() 메서드가 호출된다.
메서드는 정적인 HTML 문자열을 생성하여 클라이언트에게 반환하게 되어, 브라우저에서는 "Hello Spring Boot"라는 제목이 출력된다.
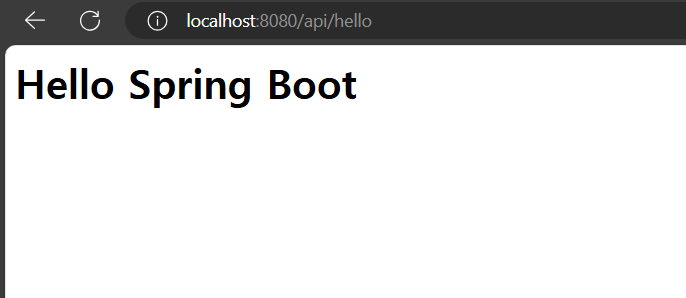
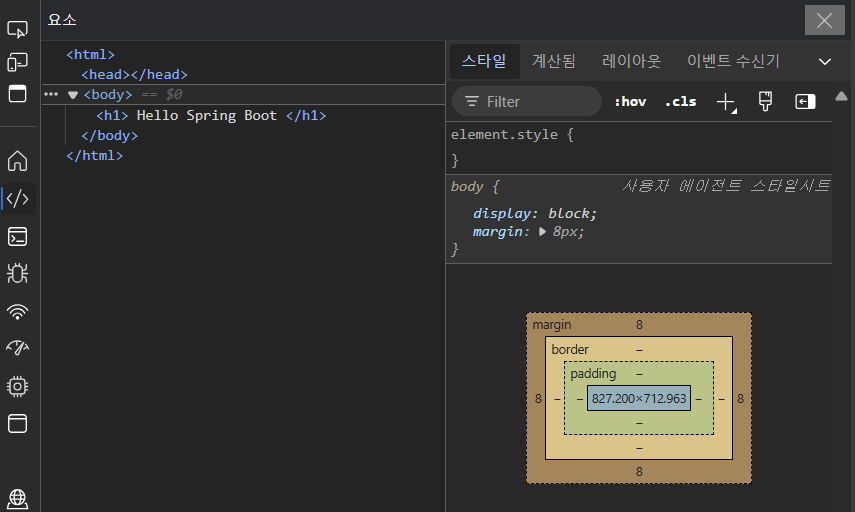
실습 3
package com.example.rest_api.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class RestApiController {
// GET 요청이 "/api/echo/{message}" 경로로 들어오면 실행되는 메서드
@GetMapping(path = "/echo/{message}")
public String echo(@PathVariable String message) {
System.out.println("echo message: " + message); // 받아온 message 값을 콘솔에 출력
return message; // 받아온 message 값을 그대로 반환
}
}
echo() 메서드는 /api/echo/{message} 경로로 GET 요청이 들어오면 {message} 경로 변수의 값이 message 매개변수로 전달된다.
예를 들어, /api/echo/hello와 같은 요청이 오면 콘솔에는 "echo message: hello"가 출력되고, 클라이언트는 "hello" 문자열을 받는다.
이를 통해 @PathVariable을 사용하여 동적인 URL 경로를 통해 데이터를 전달받고 처리할 수 있다.
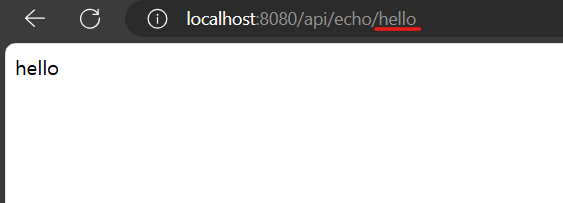
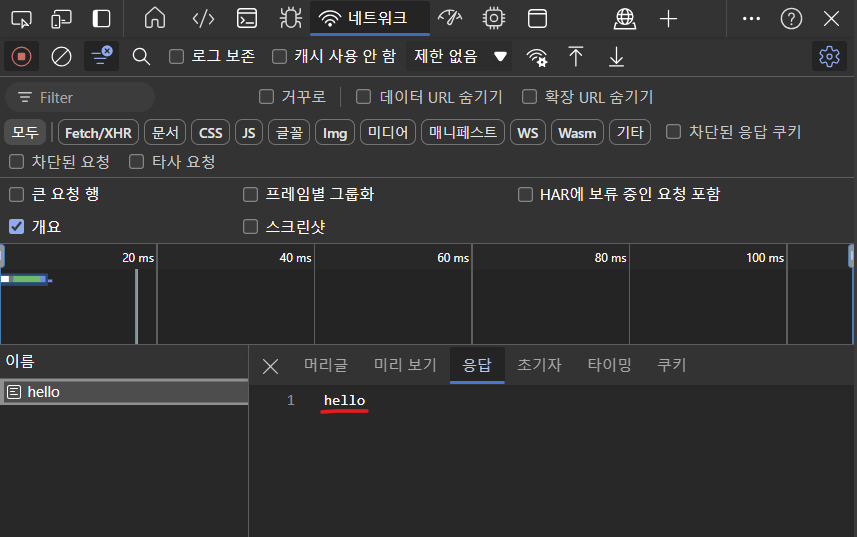

실습 4
@RestController
// GET 요청이 "/api/echo/{message}/age/{age}/is-man/{isMan}" 경로로 들어오면 실행되는 메서드
@GetMapping(path = "/echo/{message}/age/{age}/is-man/{isMan}")
public String echo(
@PathVariable(name = "message") String msg, // {message} 경로 변수를 msg 매개변수로 받음
@PathVariable int age, // {age} 경로 변수를 age 매개변수로 받음
@PathVariable boolean isMan // {isMan} 경로 변수를 isMan 매개변수로 받음
){
// 받아온 값들을 콘솔에 출력
System.out.println("echo message: " + msg);
System.out.println("echo message: " + age);
System.out.println("echo message: " + isMan);
return msg.toUpperCase(); // 받아온 message 값을 대문자로 변환하여 반환
}
}
예를 들어, /api/echo/joori/age/20/is-man/false와 같은 요청이 오면,
콘솔에는 "echo message: joori", " echo message: joori : 30", " echo message: joori : true"가 출력되고, 클라이언트는 "JOORI" 문자열을 받게 된다.
이렇게 @PathVariable을 사용하여 동적인 URL 처리를 할 수 있다.
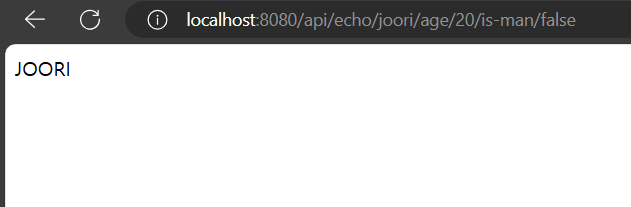
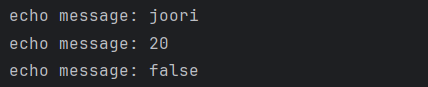
'WEB > Spring Boot' 카테고리의 다른 글
[Spring Boot] REST API - PUT (+ Talend API Tester) (0) | 2024.07.14 |
---|---|
[Spring Boot] REST API - POST (+ Talend API Tester) (0) | 2024.07.14 |
[Java] 클래스로 객체 모델링 (0) | 2024.04.09 |
[Java] 메서드(Method) - 매개변수 전달 기법, 메서드 오버로딩 (1) | 2024.04.08 |
[Java] 객체와 클래스의 이해 (1) | 2024.01.31 |
댓글